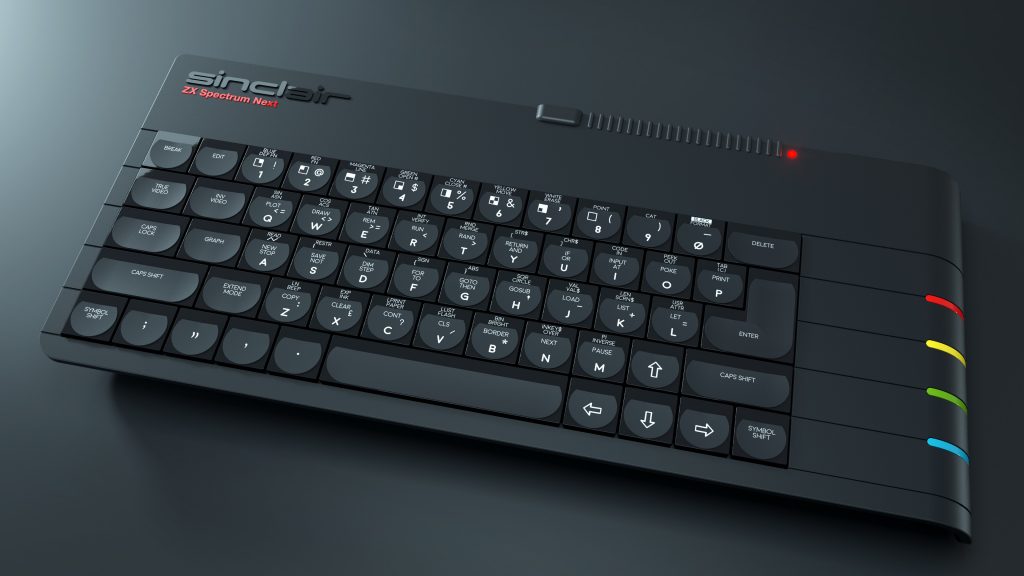
NextBASIC Inline Assembler
A downloadable z80 Assembler
ASM - ZX Spectrum Next Inline Assembler
For more detail take a look at the documentation
ASM is a .dot command that enables you to write inline assembly code in your NextBASIC application. When you invoke ASM, it scans the code for assembly instructions after the ';' symbol and assembles them into memory. You can then execute the assembled code as any machine code routine that you loaded or poked into memory.
To set up the assembler, you need to copy the 'ASM' file into the "dot" folder in the root of your ZX Spectrum Next SD card. That's all you need to do to get started.
To test the assembler, enter the following program in the NextBASIC editor. NOTE: You must have at least one space after the ';' symbol in lines 30-50. This is explained in more detail later.
10 CLEAR $bfff : REM Reserve memory for our machine code 20 .asm 30 ; org $c000 40 ; ld bc, 42 50 ; ret 60 PRINT "BC="; USR ($c000)
Run the program and you should see BC=42 printed on the screen. You can change the value in line 40 to something else and run the program again to see the output change.
How does it work?
When you run ASM, it looks at the next line of code in your program and checks if it starts with a ';'. If it does, it tries to assemble the instructions after the ';'. It repeats this process for each line until it finds a line that does not start with a ';'.
Why are the spaces important?
The assembler lets you define your own labels, which are names for memory locations or constants. A label must start right after the ';' symbol and be at most 11 characters long. To tell the assembler that you are not defining a label, you need to put a space before any instruction that is on its own line.
Examples of Labels
This section shows how to use labels in assembly language. Labels are names that represent addresses or values in memory. They make the code easier to read and maintain.
Example 1: Using a Label as a Constant
This example declares a label count
as a constant, which is referenced in the code. The assembler can handle the fact that the label is defined later in the code. Notice that when we declare count
, it is backed up right against the ;
.
10 CLEAR $bfff : REM Reserve memory for our machine code 20 .asm 30 ; org $c000 40 ; ld bc, count 50 ; ret 60 ;count equ 6144 70 PRINT "BC="; USR ($c000)
Example 2: Using a Label as a Jump Target
And here it is, our "Hello World" program
10 CLEAR $bfff : REM Reserve memory for our machine code 20 .asm 30 ; org $c000 40 ; ld a, 2 50 ; call $1601 ;Open chan 2 60 ; ld hl, msg 70 ;nxtch 80 ; ld a, (hl) 90 ; or a 100 ; ret z 110 ; rst 16 120 ; inc hl 130 ; jr nxtch 140 ;msg db "Hello world",0 150 RANDOMIZE USR ($c000)
Features of the Assembler
This section describes the features of the assembler, such as the supported instructions, pseudo-ops, and expressions.
Supported Instructions
The assembler implements all the documented Z80 and Z80N instructions, but they are far from tested.
The Z80n instructions support both the long form and the 4 character short form of the opcodes.
Using the BANK pseudo-op you can target code generation to a specific 16K bank
Supported Pseudo-Ops
The assembler supports the following pseudo-ops:
$
- Current program counter#
- Temporarily turn off relocation for the expression that it precedesALIGN
- Align code/data on the specified byte boundaryASSERT
- Asserts that an expression is true (non-zero), a false expression will raise an assertion errorBANK
- The assembled code is placed in a target bank, and optionally at an offset from the beginning of the bankBRK
- Inserts a CSpect break point in the codeDB
- Defines a byte, a list of bytes, or a double-quoted stringDC
- Defines a string or list of strings, where the last character of each string has the high bit setDS
- Defines a block of memoryDW
- Defines a 2-byte word or a list of words, or a double-quoted stringDZ
- Defines a string or list of strings, where each string is terminated by a null terminatorDISPLAY
- Output strings and expressions during assemblyEQU
- Defines a constantIF/ELSE/END
- Conditional assembly
INCLUDE
- Include external source files in the current assembly source
LET
- Assign a value to a NextBASIC integer variableNBRK
- Inserts a hardware break point in the code (nextreg $02, $08)ORG
- The ORG directive sets the expected location of your code in memory. If you omit it, the assembler will assume a location of $c000 (49152)OUTPUT
- Direct the assembled code to a fileRELOC_START/RELOC_END/RELOC_COUNT/RELOC_TABLE
- Support relocatable code, specifically for NextZXOS DriversSAVENEX
- Create a NEX file from the assembled codeVAR
- Defines a variable label that can change value between assembly phases/passes
After specifying a target bank, the assembler resets ORG to $c000. Specifying ORG after BANK will not affect the target location of the code, but can be used to affect the address calculations.
BANK 40 ; Assemble to bank 40 starting at offset 0 BANK 40, 128 ; Assemble to bank 40 starting at offset 128
In both of the above cases, the assembler assumes that when the banked code is paged in it will be paged in at address $c000. So all addresses will be calculated using that as the base. The following will direct the assembler to assemble to BANK 40 using $e000 as the base for address calculations
BANK 40 ORG $e000
To execute code generated/loaded to bank 40 at offset 128 you would do the following in NextBASIC
RANDOMIZE BANK 40 USR 128
Supported Expressions
Numeric literal can be written in decimal, hexadecimal and binary form.
Hexadecimal and binary representations can be entered using the following prefixes:
$
- Hexadecimal eg. $c000, $f3% or @
- Binary eg. %1101101 or @1101101
The following operators are supported:
+
- Addition (Also unary plus)-
- Subtraction (Also unary minus)*
- Multiplication/
- Division%
- Remainder<
- Less than<=
- Less than or equal>
- Greater than>=
- Greater than or equal=
- Equal<>
- Not equal&
- Bitwise AND^
- Bitwise XOR|
- Bitwise OR~
- Bitwise NOT (1s complement)&&
- Logical AND||
- Logical OR<<
- Left shift>>
- Right shift
Future Improvements
This section lists some possible improvements for the assembler in the future.
- Reduce the size of the assembler so there is space for more features
- Support passing a file name to the command so that it can assemble a source file into memory
Support more operators and add operator precedenceShift the symbol table to a separate bankSupport assembling code into a target bank
Please report any issues using the github issue tracker
Status | In development |
Category | Tool |
Rating | Rated 5.0 out of 5 stars (5 total ratings) |
Author | taylorza |
Tags | assembler, machine-code, nextbasic, z80, z80n, ZX Spectrum |
Download
Click download now to get access to the following files: